How to evaluate the quality and maintainability of a template
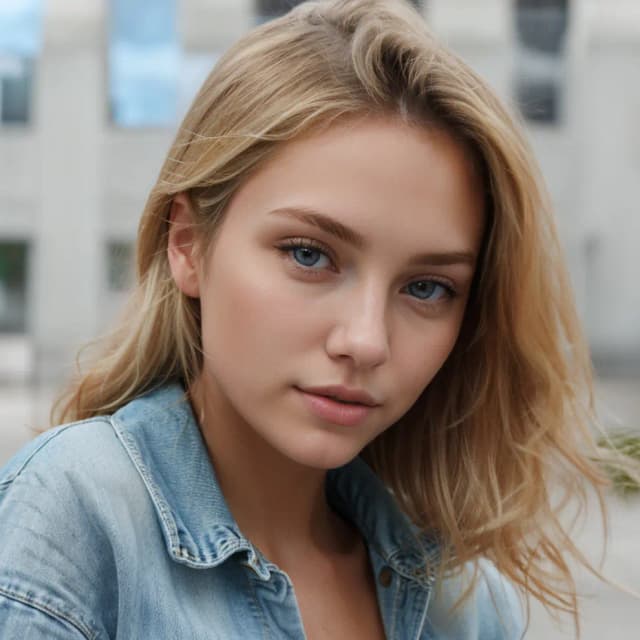
Templates can be a huge time-saver. They provide a starting point for your project, allowing you to focus on customization and unique features rather than building everything from scratch. However, not all templates are created equal. Choosing the right template can set you up for success, while a poor choice can lead to headaches down the road. In this article, we'll explore how to evaluate the quality and maintainability of a template, ensuring you make the best choice for your project.
1. Introduction
The importance of choosing the right template cannot be overstated. A well-designed, maintainable template can significantly impact your project's success and long-term sustainability. It can speed up development, improve code quality, and make it easier to add features or fix bugs in the future. On the other hand, a poorly structured or outdated template can lead to technical debt, performance issues, and difficulties in scaling your project.
2. Code Structure and Organization
One of the first things to look at when evaluating a template is its code structure and organization. A well-organized template will make it easier to understand, modify, and maintain the code.
Folder Structure and File Naming Conventions
Look for a logical and intuitive folder structure. Files should be organized in a way that makes sense, with clear separation between different types of components, styles, and functionality.
File naming should be consistent and descriptive. For instance, component files might follow a pattern like ComponentName.tsx for TypeScript projects.
Modularity and Separation of Concerns
The template should demonstrate good separation of concerns.
export default function UserProfile({ userId }) {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
fetch(`/api/users/${userId}`)
.then(res => res.json())
.then(data => {
setUser(data);
setLoading(false);
})
.catch(err => {
setError(err);
setLoading(false);
});
}, [userId]);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>{user.name}</h1>
<p>Email: {user.email}</p>
<p>Bio: {user.bio}</p>
</div>
);
}
This means that different parts of the application should be responsible for distinct functionalities. For example, UI components should be separate from business logic, and data fetching should be isolated from rendering.
import { useUser } from '../hooks/useUser';
import UserProfileView from './UserProfileView';
import LoadingSpinner from './LoadingSpinner';
import ErrorMessage from './ErrorMessage';
export default function UserProfile({ userId }) {
const { user, loading, error } = useUser(userId);
if (loading) return <LoadingSpinner />;
if (error) return <ErrorMessage message={error.message} />;
return <UserProfileView user={user} />;
}
Use of Design Patterns and Architectural Principles
Look for the use of established design patterns and architectural principles. For instance, in a React project, you might look for the use of the Container/Presentational pattern, or in a larger application, you might look for a clear implementation of state management (e.g., Redux, MobX, or React Context).
3. Code Quality
The quality of the code in a template is crucial. It affects not only the initial development but also long-term maintenance and scalability.
Readability and Consistency
The code should be easy to read and understand. Look for consistent formatting, meaningful variable names, and functions that follow the single responsibility principle.
Here's an example of extreme poor vs. good readability:
Poor readability:
function x(a,b){let c=0;for(let i=0;i<a.length;i++){if(a[i]>b){c++}}return c}
Good readability:
function countElementsGreaterThan(array, threshold) {
let count = 0;
for (let element of array) {
if (element > threshold) {
count++;
}
}
return count;
}
4. Performance Considerations
Performance is a critical factor in modern web development. A good template should be optimized for performance out of the box.
Initial Load Time and Bundle Size
Check the initial bundle size of the template. Smaller bundle sizes generally lead to faster load times. Tools like Webpack Bundle Analyzer can help you visualize what's contributing to the bundle size.
Efficient Use of Resources
Look at how the template manages resources. Are there unnecessary re-renders in React components? Is DOM manipulation minimized? These factors can significantly impact the performance of your application.
Lazy Loading and Code Splitting Implementation
For larger applications, check if the template implements lazy loading and code splitting. Here's an example of how this might be implemented in a React application:
import React, { Suspense, lazy } from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import LoadingSpinner from './components/LoadingSpinner';
const Home = lazy(() => import('./pages/Home'));
const About = lazy(() => import('./pages/About'));
const Contact = lazy(() => import('./pages/Contact'));
export default function App() {
return (
<Router>
<Suspense fallback={<LoadingSpinner />}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Suspense>
</Router>
);
}
This example uses React's `lazy` and `Suspense` to implement code splitting and lazy loading, which can significantly improve initial load times for large applications.
5. Scalability
A good template should be able to grow with your project.
Ability to Add New Features Without Major Refactoring
The structure of the template should allow for easy addition of new features. This often means having a modular architecture where new components or modules can be added without affecting existing ones.
Support for Different Screen Sizes and Devices
In today's multi-device world, responsive design is crucial. Check if the template includes responsive styles and if it's easy to adapt the layout for different screen sizes.
Internationalization and Localization Readiness
Even if your project doesn't immediately need multiple language support, it's good to choose a template that's ready for internationalization. This might include the use of translation libraries or the separation of text content from components.
6. Dependency Management
The way a template manages its dependencies can have a big impact on its long-term maintainability.
Up-to-date Dependencies
Check if the template's dependencies are up-to-date. Using outdated packages can lead to security vulnerabilities and missed performance improvements.
Minimal Use of Third-party Libraries
While third-party libraries can save development time, too many dependencies can bloat your project and introduce potential security risks. A good template should strike a balance, using third-party libraries for complex functionalities but implementing simpler features directly.
Clear Documentation of Dependencies and Their Purposes
The template should clearly document what each dependency is used for. This makes it easier to remove or replace dependencies if needed in the future.
{
"name": "my-template",
"version": "1.0.0",
"dependencies": {
"react": "^19.0.0",
"react-dom": "^19.0.0",
},
"devDependencies": {
"@types/react": "^19.0.0-rc",
"@types/react-dom": "^19.0.0-rc",
"typescript": "^5.6.8"
}
}
7. Testing and Quality Assurance
A well-maintained template should include some level of testing.
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Button from './Button';
test('calls onClick prop when clicked', () => {
const handleClick = jest.fn();
const { getByText } = render(<Button onClick={handleClick}>Click me</Button>);
fireEvent.click(getByText(/click me/i));
expect(handleClick).toHaveBeenCalledTimes(1);
});
Presence of Unit Tests and Integration Tests
Look for the presence of unit tests for individual components and functions, as well as integration tests for how different parts of the application work together.
Code Coverage
Check the code coverage of the tests. While 100% coverage isn't always necessary or practical, a good template should have substantial test coverage for critical parts of the application.
Continuous Integration/Continuous Deployment (CI/CD) Setup
A CI/CD setup can help maintain code quality over time. Check if the template includes configuration for CI/CD tools like GitHub Actions, Travis CI, or CircleCI.
8. Security Considerations
Security should be a priority in any web application.
Implementation of Security Best Practices
Look for implementation of security best practices such as input validation, output encoding, and proper authentication and authorization mechanisms.
Handling of Sensitive Data
Check how the template handles sensitive data. Are there clear patterns for managing things like API keys or user credentials?
Regular Security Updates
Check if the template is regularly updated to address security vulnerabilities, especially in its dependencies.
9. Accessibility
Accessibility is increasingly important in web development, both for usability and legal compliance.
export default function AccessibleForm() {
return (
<form>
<div>
<label htmlFor="name">Name:</label>
<input
type="text"
id="name"
name="name"
aria-required="true"
aria-describedby="name-error"
/>
<span id="name-error" className="error" aria-live="polite"></span>
</div>
<div>
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
name="email"
aria-required="true"
aria-describedby="email-error"
/>
<span id="email-error" className="error" aria-live="polite"></span>
</div>
<button type="submit">Submit</button>
</form>
);
}
Compliance with WCAG Guidelines
Check if the template follows Web Content Accessibility Guidelines (WCAG). This includes things like proper color contrast and keyboard navigation support.
Semantic HTML Usage
Look for the use of semantic HTML elements. This not only improves accessibility but also helps with SEO.
Keyboard Navigation Support
Ensure that all interactive elements can be accessed and used with a keyboard.
10. Community and Support
The community around a template can be a valuable resource.
Active Maintenance and Updates
Check when the template was last updated. An actively maintained template is more likely to stay current with best practices and security updates.
Size and Engagement of the Community
Look at factors like the number of stars on GitHub, frequency of issues and pull requests, and how quickly they're addressed. A larger, more engaged community can be a great source of support and improvements.
Quality of Documentation and Examples
Good documentation can save you a lot of time and frustration. Look for clear, comprehensive documentation with examples of how to use and extend the template.
11. Customization and Flexibility
A good template should be a starting point, not a rigid structure.
Ease of Modifying Styles and Layouts
Check how easy it is to change the look and feel of the template. Are styles well-organized and easy to override?
Ability to Add or Remove Features
The template should make it easy to add new features or remove ones you don't need without breaking other parts of the application.
Clear Extension Points for Custom Functionality
Look for clear patterns or documentation on how to extend the template with your own custom functionality.
12. Compatibility
Ensure the template works across different environments.
Browser Compatibility
Check which browsers the template supports. It should work well in all major modern browsers.
Support for Different Devices and Operating Systems
The template should work well on different devices (desktop, tablet, mobile) and operating systems.
Integration with Popular Tools and Frameworks
If you're planning to use specific tools or frameworks, check if the template integrates well with them or provides examples of how to integrate them.
13. Performance in Real-World Scenarios
Look beyond the demo and consider real-world performance.
Case Studies or Examples of Successful Implementations
If available, look for case studies or examples of projects that have successfully used the template. This can give you an idea of how it performs in production environments.
Benchmarks Against Similar Templates
If possible, compare the performance of the template against similar options. This can help you understand its strengths and weaknesses.
14. Tools and Techniques for Evaluation
There are several tools that can help you evaluate a template:
Code analysis tools like ESLint can help you assess code quality.
Performance profiling tools like Lighthouse can give you insights into performance.
Accessibility checkers like axe can help you evaluate accessibility compliance.
15. Conclusion
Choosing the right template is a crucial decision that can significantly impact your project's success. By carefully evaluating the quality and maintainability of a template across these various factors, you can make an informed decision that sets your project up for success.
Remember, no template will be perfect for every situation. The key is to find one that aligns well with your project's needs and provides a solid foundation that you can build upon. Don't be afraid to invest time in this evaluation process - it can save you countless hours of refactoring and bug-fixing down the line.
Happy coding!